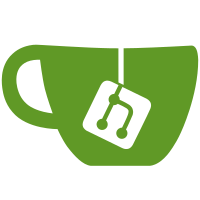
- /html/about.php - /html/admin.php - /html/compte.php - /html/editpage.php - /html/login.php - /html/logout.php - /html/news.php - /html/register.php - /html/src/banner/index.php - /html/src/img/empty.jpg - /html/src/img/favicon.ico - /html/src/miniature/index.php - /html/src/pp/index.php - /html/upload.php - /html/user.php - /html/users.php - /content/journal/0.md - /content/journal/198183.md - /content/about.md - /content/admin.md - /content/index.md - /html-old/editpage.php - /html-old/src/banner/index.php - /html-old/src/css/index.php - /html-old/src/css/style.css - /html-old/src/fonts/index.php - /html-old/src/fonts/bahnschrift.ttf - /html-old/src/img/empty.jpg - /html-old/src/img/favicon.ico - /html-old/src/img/index.php - /html-old/src/img/athena-mono.png - /html-old/src/miniature/index.php - /html-old/src/pp/index.php - /html-old/src/index.php - /html-old/about.php - /html-old/robots.txt - /html-old/admin.php - /html-old/compte.php - /html-old/login.php - /html-old/logout.php - /html-old/index.php - /html-old/news.php - /html-old/register.php - /html-old/upload.php - /html-old/users.php - /html-old/user.php - /html/about/index.php - /html/account/index.php - /html/admin/index.php - /html/assets/banners/index.php - /html/assets/miniatures/index.php - /html/assets/pp/index.php - /html/assets/index.php - /html/editor/index.php - /html/login/index.php - /html/login/logout.php - /html/login/register.php - /html/news/index.php - /html/settings/index.php - /html/settings/deleteaccount.php - /html/src/css/style.css - /html/src/img/athena-mono.png - /html/index.php - /html/robots.txt - /content/articles/0.md - /content/articles/198183.md - /content/pages/about.md - /content/pages/admin.md - /content/pages/index.md - /include/variables.php - /include/functions.php - /config/global.ini
200 lines
7.4 KiB
PHP
200 lines
7.4 KiB
PHP
<?php
|
|
require("../../include/variables.php");
|
|
require("../../include/functions.php");
|
|
$pdo = sqlConnect($sqlDatabaseHost, $sqlDatabaseName, $sqlDatabaseUser, $sqlDatabasePass);
|
|
|
|
if ($_SESSION['level'] < 1) {
|
|
header("Location: login.php");
|
|
http_response_code(404);
|
|
}
|
|
|
|
// Getting article informations
|
|
|
|
if (isset($_GET['article'])) {
|
|
$sqlRequest = "SELECT ID, title, resume, miniature, classification FROM articles WHERE ID = :articleID AND author = :authorID";
|
|
$request = $pdo->prepare($sqlRequest);
|
|
$request->bindParam(":articleID", $_GET['article']);
|
|
$request->bindParam(":authorID", $_SESSION['userID']);
|
|
$request->execute();
|
|
$result = $request->fetchAll(PDO::FETCH_ASSOC);
|
|
if ($result) {
|
|
$articleID = $result[0]['ID'];
|
|
$articleTitle = $result[0]['title'];
|
|
$articleResume = $result[0]['resume'];
|
|
$articleClassification = $result[0]['classification'];
|
|
$miniatureURL = $result[0]['miniature'];
|
|
$articleContent = file_get_contents($rootFilePath . 'content/articles/' . $articleID . '.md');
|
|
} else {
|
|
$status = "Article introuvable";
|
|
}
|
|
} else {
|
|
$status = "Veuillez choisir un article";
|
|
}
|
|
|
|
|
|
// Article deletion
|
|
|
|
if (isset($_POST['delete-article']) && $_POST['delete-article'] == "delete") {
|
|
$sqlRequest = "DELETE FROM articles WHERE ID = :articleID";
|
|
$request = $pdo->prepare($sqlRequest);
|
|
$request->bindParam(":articleID", $articleID);
|
|
if($request->execute()) {
|
|
$status = "Article supprimé";
|
|
$articleTitle = "";
|
|
$articleResume = "";
|
|
$articleContent = "";
|
|
$miniatureURL = "";
|
|
}
|
|
}
|
|
|
|
|
|
// Updating the article after upload
|
|
|
|
if (isset($_POST['article-content']) && isset($_POST['classification']) && isset($articleID)) {
|
|
|
|
file_put_contents($rootFilePath . "content/articles/" . $articleID . ".md", nl2br($_POST['article-content']));
|
|
|
|
$sqlRequest = "UPDATE articles SET title = :title, resume = :resume, classification = :classification WHERE ID = :articleID AND author = :authorID";
|
|
$request = $pdo->prepare($sqlRequest);
|
|
$request->bindParam(":title", htmlspecialchars($_POST['article-title']));
|
|
$request->bindParam(":resume", htmlspecialchars($_POST['article-resume']));
|
|
$request->bindParam(":classification", $_POST['classification'], PDO::PARAM_INT);
|
|
$request->bindParam(":articleID", $_GET['article'], PDO::PARAM_INT);
|
|
$request->bindParam(":authorID", $_SESSION['userID'], PDO::PARAM_INT);
|
|
$request->execute();
|
|
$result = $request->fetchAll(PDO::FETCH_ASSOC);
|
|
if($request->execute()) {
|
|
header("Location: /editor?article=" . $articleID);
|
|
} else {
|
|
$status = "Une erreur s'est produite";
|
|
}
|
|
}
|
|
|
|
|
|
// Updating the miniature
|
|
|
|
if (isset($_FILES['miniature']) && $_FILES['miniature']['error'] == 0 && isset($articleID)) {
|
|
$tempImagePath = $_FILES['miniature']['tmp_name'];
|
|
$ImagePath = 'assets/miniatures/' . $articleID . '.png';
|
|
$rootImagePath = $rootFilePath . 'html/' . $ImagePath;
|
|
$imageURL = $rootPageURL . $ImagePath;
|
|
|
|
list($width, $height) = getimagesize($tempImagePath);
|
|
|
|
$imageInfo = getimagesize($tempImagePath);
|
|
|
|
if ($imageInfo[2] === IMAGETYPE_PNG || $imageInfo[2] === IMAGETYPE_JPEG) {
|
|
$imageWidth = 500;
|
|
$imageHeight = ($height / $width) * $imageWidth;
|
|
$imageResized = imagecreatetruecolor($imageWidth, $imageHeight);
|
|
$imageOriginal = imagecreatefromstring(file_get_contents($tempImagePath));
|
|
|
|
imagecopyresampled($imageResized, $imageOriginal, 0, 0, 0, 0, $imageWidth, $imageHeight, $width, $height);
|
|
imagealphablending($imageResized, false);
|
|
imagesavealpha($imageResized, $rootPath);
|
|
|
|
imagepng($imageResized, $rootImagePath);
|
|
|
|
imagedestroy($imageOriginal);
|
|
imagedestroy($imageResized);
|
|
|
|
$sqlRequest = "UPDATE articles SET miniature = :miniature WHERE ID = :articleID";
|
|
$request = $pdo->prepare($sqlRequest);
|
|
$request->bindParam(":miniature", $imageURL);
|
|
$request->bindParam(":articleID", $articleID);
|
|
if($request->execute()) {
|
|
$status = "Miniature changée";
|
|
$miniatureURL = $imageURL;
|
|
} else {
|
|
$status = "Erreur SQL";
|
|
}
|
|
} else {
|
|
$status = "Le fichier doit être au format PNG ou JPG";
|
|
}
|
|
}
|
|
|
|
?>
|
|
|
|
<!DOCTYPE html>
|
|
<html lang="fr">
|
|
|
|
<head>
|
|
<?php fillHead($rootPageURL, $pageTitle, $darkTheme, $lightTheme);?>
|
|
<style>
|
|
.text-input label {
|
|
width: 0;
|
|
}
|
|
textarea {
|
|
min-width: 80%;
|
|
}
|
|
.article-content-input {
|
|
min-height: 40em;
|
|
}
|
|
.miniature-change-zone {
|
|
margin-top: 5em;
|
|
}
|
|
.image-input {
|
|
display: flex;
|
|
gap: 1em;
|
|
}
|
|
.miniature-preview img {
|
|
width: 20em;
|
|
border-radius: 0;
|
|
}
|
|
#delete-button {
|
|
margin-top: 5em;
|
|
}
|
|
</style>
|
|
</head>
|
|
|
|
<body class="body">
|
|
|
|
<header>
|
|
<div class="panel-content">
|
|
<?php fillHeader($rootPageURL, $headerTitle, $headerSubtitle);?>
|
|
</div>
|
|
</header>
|
|
|
|
<nav>
|
|
<div class="panel-content">
|
|
<?php fillNav($rootPageURL);?>
|
|
</div>
|
|
</nav>
|
|
|
|
<main>
|
|
<div class="content">
|
|
<div class="status"><?=$status?></div>
|
|
<form action="?article=<?=$articleID?>" method="post">
|
|
<div>
|
|
<div><h1>Editer un article</h1></div>
|
|
<?php textInput("text", "", "article-title", "Titre de l'article", $articleTitle)?>
|
|
<div><textarea name="article-resume" placeholder="Résumé de l'article (200 cacactères) ..." class="article-resume-input" maxlength="200"><?=$articleResume?></textarea></div>
|
|
<div><textarea name="article-content" placeholder="Contenu de l'article (MarkDown) ..." class="article-content-input"><?=str_replace("<br />", "", $articleContent)?></textarea></div>
|
|
<?php
|
|
selectInput("classification", "Classification", $confidentialLevels, $articleClassification);
|
|
?>
|
|
<div><button type="submit">Publier</button></div>
|
|
</div>
|
|
</form>
|
|
<div class="miniature-change-zone">
|
|
<div class="miniature-preview"><img src="<?=$miniatureURL?>"></div>
|
|
<form action="?article=<?=$articleID?>" method="post" enctype="multipart/form-data">
|
|
<div class="image-input">
|
|
<?php fileInput("miniature")?>
|
|
<input type="submit" value="Changer la miniature" class="button">
|
|
</div>
|
|
</form>
|
|
<form action="?article=<?=$articleID?>" method="post" enctype="multipart/form-data">
|
|
<input type="hidden" name="delete-article" value="delete">
|
|
<button type="submit" id="delete-button">Supprimer l'article</button>
|
|
</form>
|
|
</div>
|
|
</div>
|
|
</main>
|
|
<footer>
|
|
<div class="panel-content">
|
|
<?php fillFooter($footerText);?>
|
|
</div>
|
|
</footer>
|
|
</body>
|
|
</html>
|